MidiFileAsset
👉 Now Available at Fab
👉 日本語版はこちら!
Introduction
MidiFileAsset is a plugin for Unreal Engine that provides the following features:
Here is a image of provided Blueprint nodes:
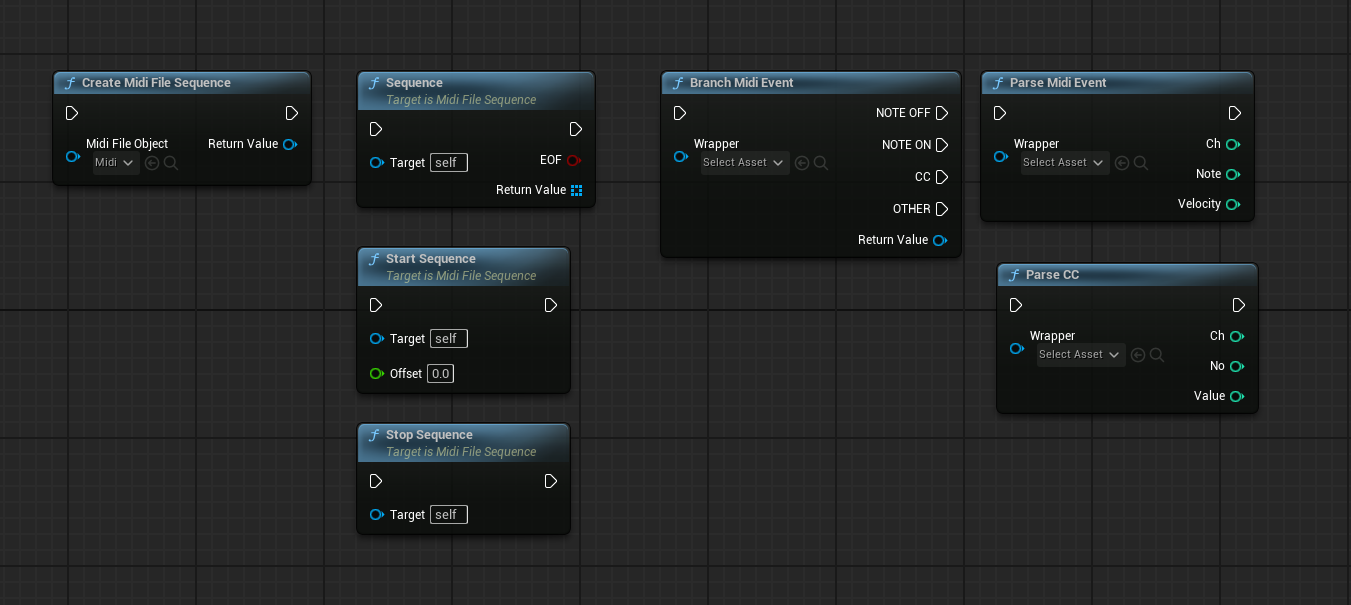
Below is a sample Blueprint that demonstrates how to load a MIDI file and output MIDI message information.
In this sample, MIDI messages played in each frame are output. The MIDI sequence can be played with the z
key and stopped with the x
key.
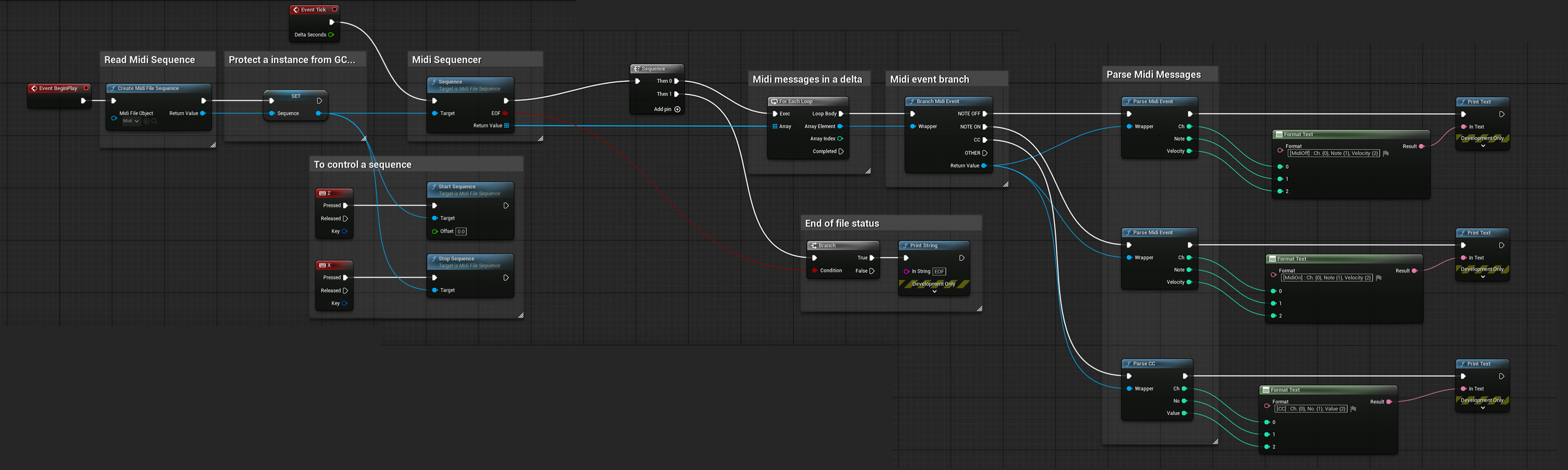
Loading MIDI Files
To load a MIDI file, drag and drop the MIDI file into the Content Browser.
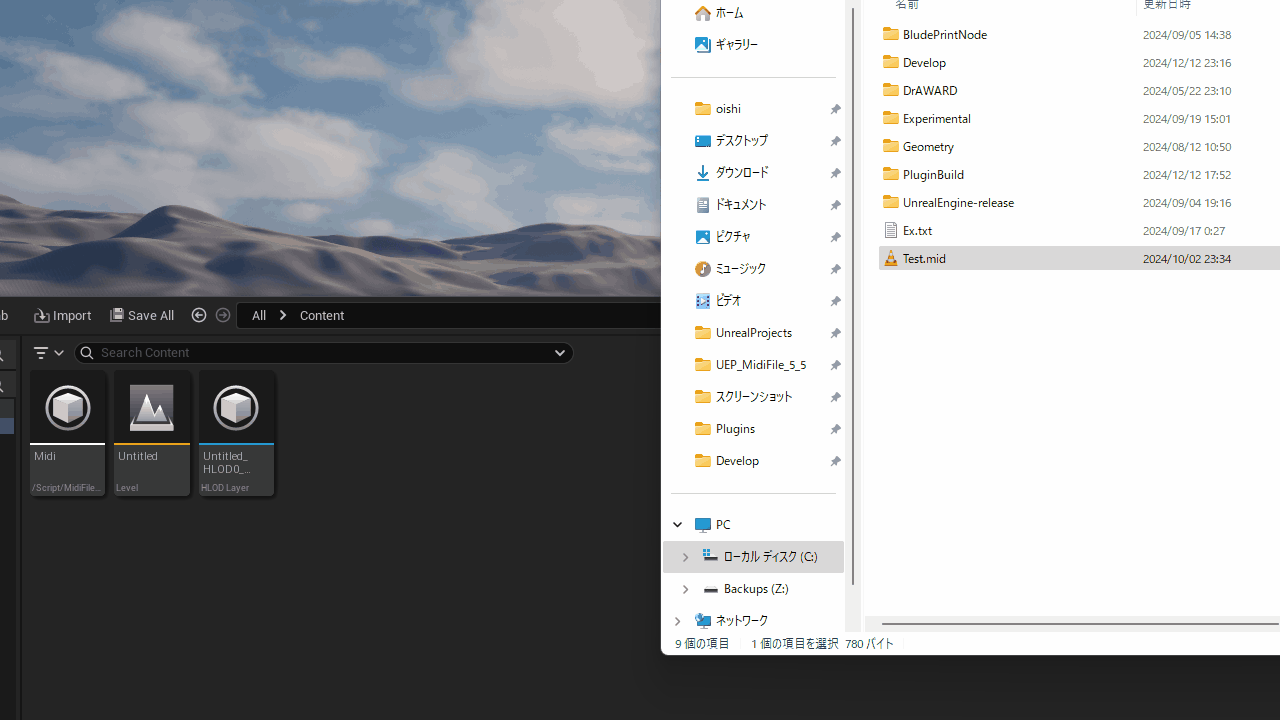
Using Blueprint Nodes
To use these nodes in a Blueprint, search for Midi File Asset
in the search bar. The relevant nodes will appear in the list.
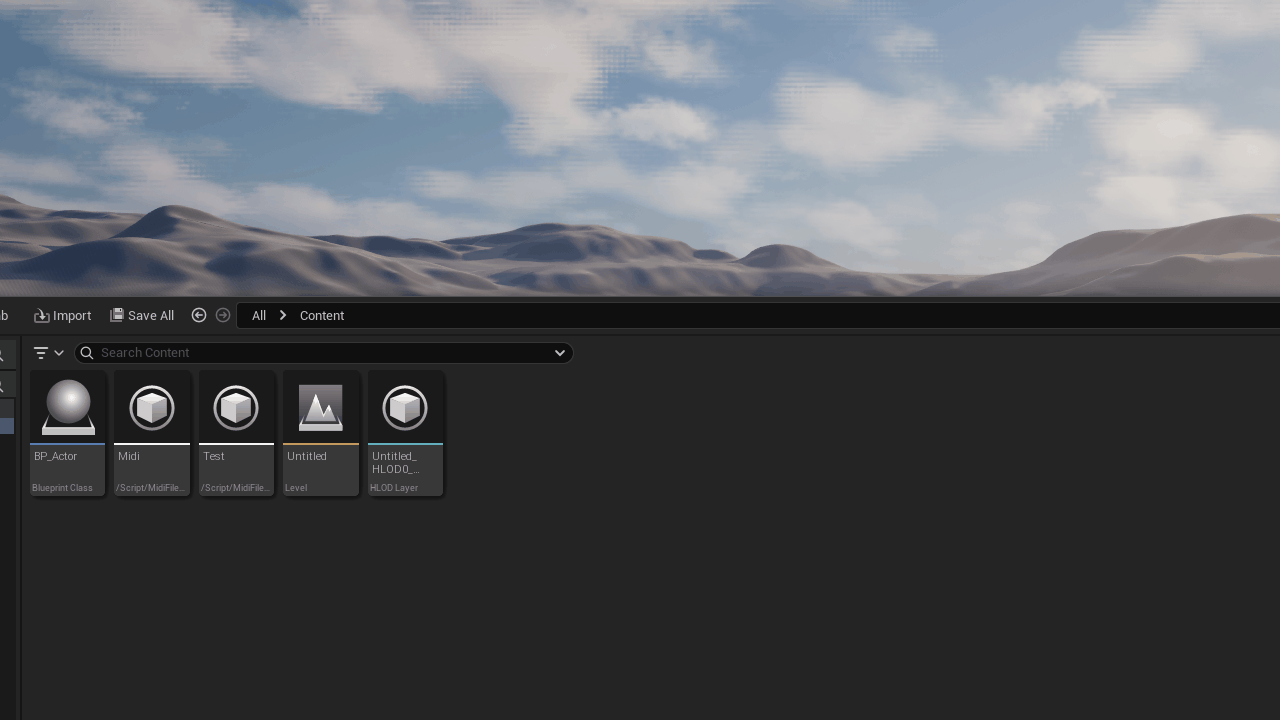
Alternatively, searching from the Return Value
can also make the nodes easier to find.
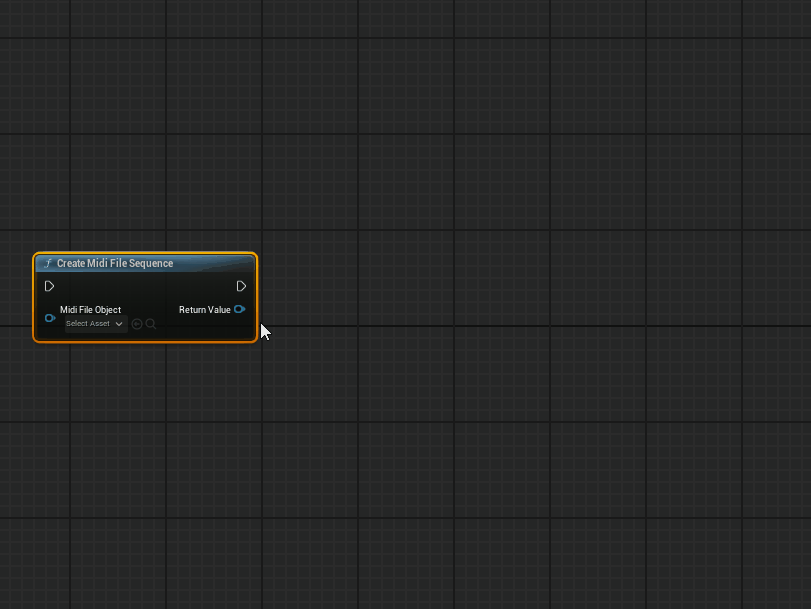
Blueprint Node Specifications
Below is the detailed specification of each provided Blueprint node.
Create Midi File Sequence
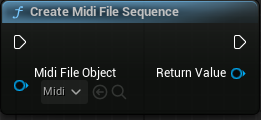
Input
- Midi File Object
- Select the loaded MIDI file from the dropdown menu.
- Execution Pin
- Creates a MIDI sequence from the input
Midi File Object
.
Output
- Return Value
- Outputs the created MIDI sequence on success.
- Execution Pin
- Triggers after the sequence creation process is complete.
Sequence
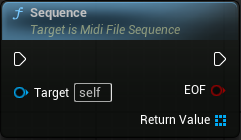
Input
- Target
- Connect the MIDI sequence created by
Create Midi File Sequence
.
- Execution Pin
- Outputs the corresponding MIDI message if the sequence is playing when triggered.
Output
- Return Value
- Outputs the current MIDI message if the sequence is playing.
- EOF
- Outputs
true
when the end of the MIDI file is reached.
- Execution Pin
- Triggers when the process within the node is complete.
Start Sequence
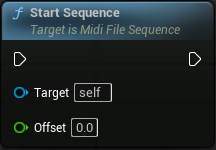
Input
- Target
- Connect the MIDI sequence created by
Create Midi File Sequence
.
- Offset
- Specifies the playback start position in seconds. Examples:
2.0
: Starts playback 2 seconds from the beginning of the file.
-2.0
: Starts playback 2 seconds after initiation.
- Execution Pin
- Starts playback of the MIDI sequence.
Output
- Execution Pin
- Triggers when the process within the node is complete.
Stop Sequence
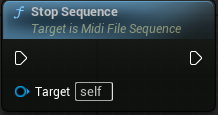
Input
- Target
- Connect the MIDI sequence created by
Create Midi File Sequence
.
- Execution Pin
- Stops playback of the MIDI sequence.
Output
- Execution Pin
- Triggers when the process within the node is complete.
Branch Midi Event
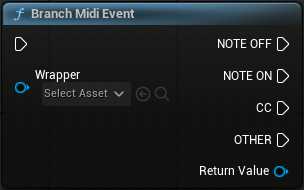
Input
- Wrapper
- Execution Pin
- Triggers different execution pins based on the type of the connected MIDI message.
Output
- NOTE OFF
- Triggers when
MidiMessage.Type == NOTE_OFF
.
- NOTE ON
- Triggers when
MidiMessage.Type == NOTE_ON
.
- CC
- Triggers when
MidiMessage.Type == CC
.
- OTHER
- Triggers for other types of messages.
- Return Value
- Outputs the input
MidiMessage
as-is.
Parse Midi Event
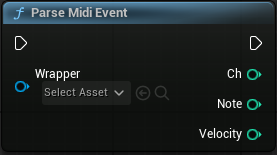
Input
- Wrapper
- Connects a
Note On
or Note Off
MidiMessage
.
- Execution Pin
- Parses the input message and outputs the following information.
Output
- Ch
- Outputs the Channel value of the message.
- Note
- Outputs the Note number value of the message.
- Velocity
- Outputs the Velocity value of the message.
- Execution Pin
- Triggers when the process within the node is complete.
Parse CC
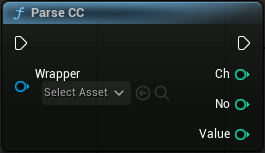
Input
- Wrapper
- Connects a
CC
type MidiMessage
.
- Execution Pin
- Parses the input Control Change message and outputs the following information.
Output
- Ch
- Outputs the Channel value of the message.
- No
- Outputs the CC number value of the message.
- Value
- Outputs the Value of the message.
- Execution Pin
- Triggers when the process within the node is complete.